What is Boto3 S3 AWS?
What is Boto3 S3 AWS. Boto3 is the name of the Python SDK for AWS. It allows you to directly create, update, and delete AWS resources from your Python scripts.
You use the AWS SDK for Python (Boto3) to create, configure, and manage AWS services, such as Amazon Elastic Compute Cloud (Amazon EC2) and Amazon Simple Storage Service (Amazon S3). The SDK provides an object-oriented API as well as low-level access to AWS services.
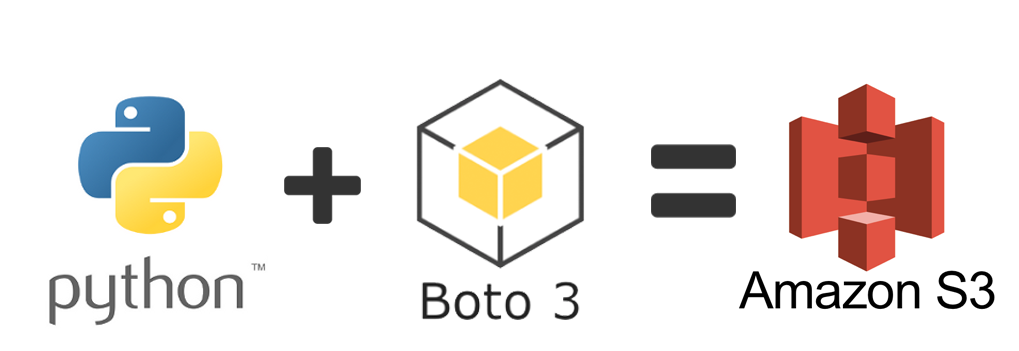
How to Create an Amazon S3 bucket Using Boto3 S3
Note
Documentation and developers tend to refer to the AWS SDK for Python as “Boto3,” and this documentation often does so as well.
For example, the name of an Amazon S3 bucket must be unique across all regions of the AWS platform. The bucket can be located in a specific region to minimize latency or to address regulatory requirements.
import logging import boto3 from botocore.exceptions import ClientError def create_bucket(bucket_name, region=None): """Create an S3 bucket in a specified region If a region is not specified, the bucket is created in the S3 default region (us-east-1). :param bucket_name: Bucket to create :param region: String region to create bucket in, e.g., 'us-west-2' :return: True if bucket created, else False """ # Create bucket try: if region is None: s3_client = boto3.client('s3') s3_client.create_bucket(Bucket=bucket_name) else: s3_client = boto3.client('s3', region_name=region) location = {'LocationConstraint': region} s3_client.create_bucket(Bucket=bucket_name, CreateBucketConfiguration=location) except ClientError as e: logging.error(e) return False return True
In addition, Amazon Web Services (AWS) has become a leader in cloud computing. One of its core components is S3, the object storage service offered by AWS. With its impressive availability and durability, it has become the standard way to store videos, images, and data. You can combine S3 with other services to build infinitely scalable applications.
How To Install Boto3?
First of all, to install Boto3 on your computer, go to your terminal and run the following:
$ pip install boto3
Basically, in order to make it run against your AWS account, you’ll need to provide some valid credentials. If you already have an IAM user that has full permissions to S3, you can use those user’s credentials (their access key and their secret access key) without needing to create a new user. Otherwise, the easiest way to do this is to create a new AWS user and then store the new credentials.
In addition, first create a new user, go to your AWS account, then go to Services and select IAM. Then choose Users and click on Add user. Furthermore, be sure to give programmatic access to the user.
What is Boto3 S3 AWS: Create AWS User
Basically, since now that you have your new user, create a new file, ~/.aws/credentials
:
$ touch ~/.aws/credentials
Thus, now open the file and paste the structure below. Fill in the placeholders with the new user credentials you have downloaded:
[default]
aws_access_key_id = YOUR_ACCESS_KEY_ID
aws_secret_access_key = YOUR_SECRET_ACCESS_KEY
Therefore,
At its core, all that Boto3 does is call AWS APIs on your behalf. For the majority of the AWS services, Boto3 offers two distinct ways of accessing these abstracted APIs:
- Client: low-level service access
- Resource: higher-level object-oriented service access
How To List Existing S3 Buckets Using Boto3
AWS Identity and Access Management (IAM) provides a way to create users that can be used to access AWS resources within one AWS account.
# Retrieve the list of existing buckets s3 = boto3.client('s3') response = s3.list_buckets() # Output the bucket names print('Existing buckets:') for bucket in response['Buckets']: print(f' {bucket["Name"]}')
Next, the users sign in to a user portal with a single set of credentials configured in AWS SSO, allowing them to access all of their assigned accounts and applications in a single place.
Hence, how to use AWS single sign on is very easy to use. Furthermore, let’s take a look at the steps.
What is Boto3 S3 AWS: How To Create AWS User Using Boto3
Firstly, Create a new IAM user for your AWS account. For information about limitations on the number of IAM users you can create, see Limitations on IAM Entities in the IAM User Guide.
For instance, Create a new IAM user using create_user.
import boto3 # Create IAM client iam = boto3.client('iam') # Create user response = iam.create_user( UserName='IAM_USER_NAME' ) print(response)
Thus, Example.com has two developers, Martha and Richard, who need full access to Amazon EC2 and Amazon S3 in the developer accounts (DevAccount1 and DevAccount2) and read-only access to EC2 and S3 resources in the production accounts (ProdAccount1 and ProdAccount2).
Diagram Representation: How To Filter Files From S3 Buckets
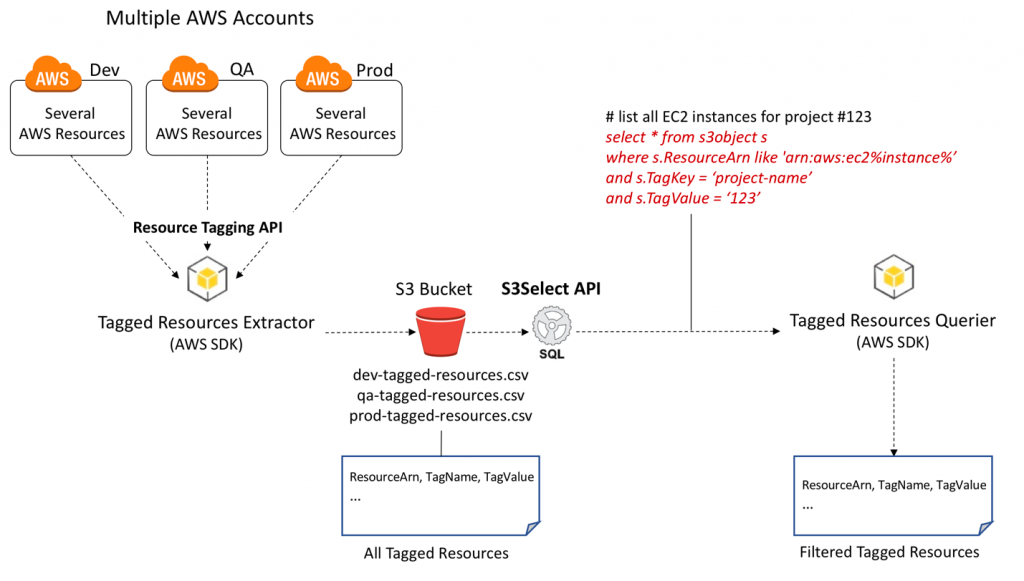

Summary
Therefore,
Before using Boto3, you need to set up authentication credentials for your AWS account using either the IAM Console or the AWS CLI. You can either choose an existing user or create a new one.
For instructions about how to create a user using the IAM Console, see Creating IAM users. Once the user has been created, see Managing access keys to learn how to create and retrieve the keys used to authenticate the user.
Thus, you have learned how to work with and understand what is Boto3 S3 AWS?